The goal for this assignment is to practice using map
and apply
.
Use map
and apply
wherever you can to implement the functions. If you can’t see how to use map
or apply
, I’ll accept other ways of implementing the functions, but do make an effort to use the higher-order functions.
Your implementations of the following functions should all be placed in a single Racket file named hw3.rkt
. The corresponding tests for each function should be in a second Racket file named tests.rkt
.
You may use the solution to any exercise as a helper function in subsequent exercises and you may also write stand-alone helper functions.
The start of each file should be
#lang racket
; Your name(s) here.
Preliminaries
Click on the assignment link. If you’re working with a partner, one partner should create a new team. The second partner should click the link and choose the appropriate team. (Please don’t choose the wrong team, there’s a maximum of two people and if you join the wrong one, you’ll prevent the correct person from joining.)
Once you have accepted the assignment and created/joined a team, you can clone the repository on your computer by following the instruction and begin working. But before you do, read the entire assignment and be sure to check out the expected coding style from the first homework.
Be sure to ask any questions on Piazza.
Submission
To submit your homework, you must commit and push to GitHub before the deadline.
Your repository should contain the following files
It may also a .gitignore
file which tells Git to ignore files matching patterns in your working directory.
Any additional files you have added to your repository should be removed from the main
branch. (You’re free to make other branches, if you desire, but make sure main
contains the version of the code you want graded.)
Make sure you put your name (and your partner’s name if you’re working with one) as a comment at the top of each file.
Finishing up
Make sure you wrote tests of all of these, the test suite for each function is included in the all-tests
test suite, and your tests pass. For reference, my solution involves 41 tests in total and when I run (test/gui all-tests)
, I get this.
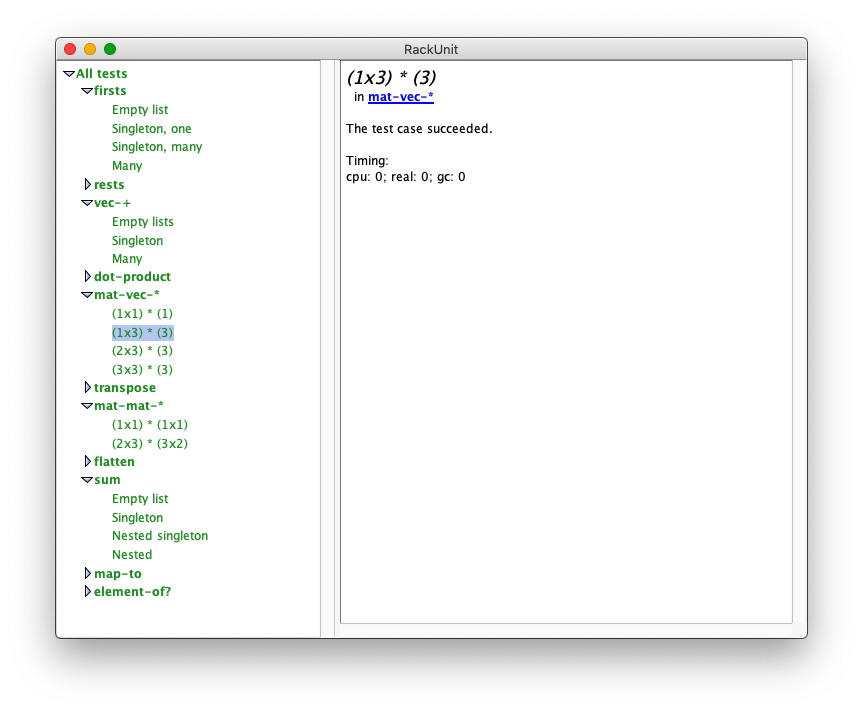
Make sure you commit and push.